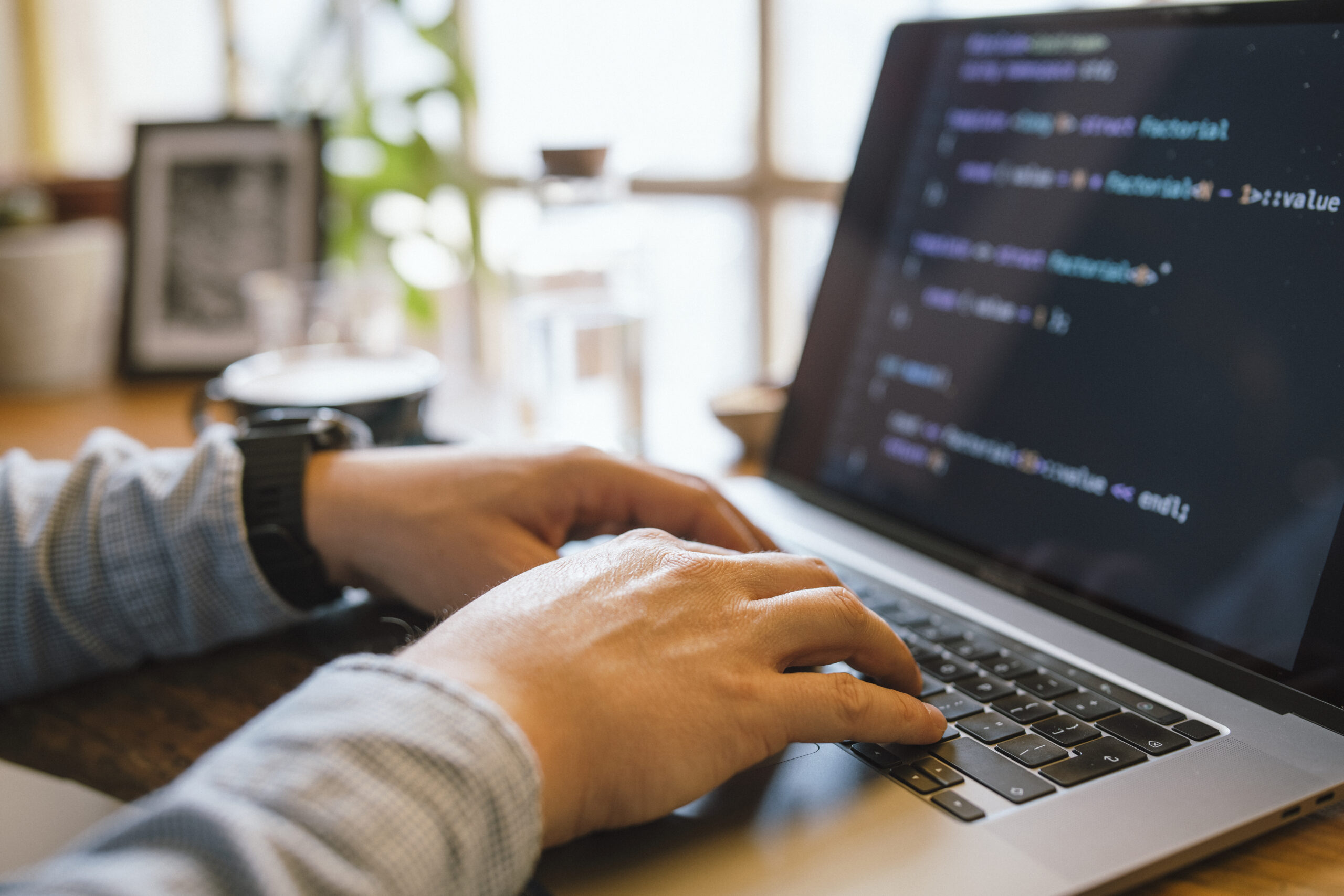
Debugging is one of the most vital — yet frequently disregarded — techniques inside of a developer’s toolkit. It's not just about fixing broken code; it’s about knowing how and why points go Completely wrong, and Discovering to think methodically to solve problems efficiently. Whether or not you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help you save several hours of irritation and radically help your efficiency. Here's various tactics that can help builders degree up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
One of the fastest means builders can elevate their debugging capabilities is by mastering the equipment they use every single day. Although creating code is 1 Component of progress, recognizing how to communicate with it properly for the duration of execution is equally important. Modern progress environments arrive equipped with impressive debugging capabilities — but numerous builders only scratch the surface area of what these tools can perform.
Consider, such as, an Built-in Development Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the worth of variables at runtime, phase through code line by line, and in many cases modify code about the fly. When used effectively, they Allow you to notice precisely how your code behaves during execution, and that is priceless for monitoring down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for entrance-stop developers. They assist you to inspect the DOM, keep an eye on network requests, watch actual-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and community tabs can change irritating UI challenges into manageable jobs.
For backend or program-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Handle about operating procedures and memory management. Finding out these tools could possibly have a steeper learning curve but pays off when debugging functionality challenges, memory leaks, or segmentation faults.
Past your IDE or debugger, develop into comfortable with Model Management units like Git to comprehend code heritage, find the exact second bugs ended up released, and isolate problematic variations.
Ultimately, mastering your tools indicates heading over and above default options and shortcuts — it’s about acquiring an personal expertise in your development atmosphere in order that when troubles occur, you’re not missing in the dead of night. The greater you already know your applications, the greater time you may shell out fixing the actual difficulty as opposed to fumbling by means of the method.
Reproduce the challenge
Among the most important — and sometimes neglected — measures in successful debugging is reproducing the issue. Before leaping in the code or generating guesses, developers need to produce a reliable setting or situation where the bug reliably seems. With no reproducibility, fixing a bug results in being a video game of possibility, frequently bringing about squandered time and fragile code modifications.
The initial step in reproducing a challenge is collecting as much context as is possible. Request concerns like: What steps resulted in The difficulty? Which setting was it in — improvement, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it will become to isolate the exact ailments below which the bug takes place.
After you’ve gathered adequate information, try and recreate the problem in your neighborhood environment. This might necessarily mean inputting precisely the same information, simulating very similar user interactions, or mimicking technique states. If the issue appears intermittently, look at creating automatic checks that replicate the edge situations or point out transitions concerned. These assessments don't just aid expose the situation but also avert regressions Down the road.
Occasionally, The problem may very well be atmosphere-distinct — it'd happen only on specific running units, browsers, or under certain configurations. Working with tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating these types of bugs.
Reproducing the issue isn’t only a phase — it’s a way of thinking. It necessitates patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be now midway to correcting it. With a reproducible scenario, You should use your debugging resources a lot more efficiently, examination likely fixes properly, and connect extra Evidently with all your workforce or users. It turns an summary criticism right into a concrete problem — and that’s in which developers thrive.
Browse and Have an understanding of the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when one thing goes Improper. As opposed to seeing them as frustrating interruptions, builders really should understand to treat error messages as immediate communications through the program. They frequently tell you what precisely took place, in which it happened, and in some cases even why it took place — if you understand how to interpret them.
Commence by studying the information meticulously and in comprehensive. A lot of developers, specially when underneath time stress, look at the primary line and instantly start building assumptions. But deeper from the error stack or logs may perhaps lie the real root trigger. Don’t just duplicate and paste error messages into search engines — examine and realize them to start with.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a certain file and line number? What module or purpose triggered it? These inquiries can manual your investigation and place you toward the dependable code.
It’s also practical to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically abide by predictable patterns, and Mastering to acknowledge these can dramatically increase your debugging procedure.
Some problems are imprecise or generic, and in Individuals scenarios, it’s crucial to examine the context through which the mistake occurred. Verify relevant log entries, enter values, and recent adjustments from the codebase.
Don’t overlook compiler or linter warnings either. These usually precede much larger issues and provide hints about prospective bugs.
Eventually, mistake messages are usually not your enemies—they’re your guides. Learning to interpret them appropriately turns chaos into clarity, aiding you pinpoint difficulties a lot quicker, reduce debugging time, and become a much more effective and assured developer.
Use Logging Properly
Logging is The most highly effective applications inside of a developer’s debugging toolkit. When used effectively, it provides real-time insights into how an software behaves, helping you understand what’s happening underneath the hood without having to pause execution or move through the code line by line.
A good logging strategy starts with knowing what to log and at what amount. Popular logging concentrations include things like DEBUG, Details, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information all through enhancement, Details for standard activities (like productive begin-ups), Alert for probable troubles that don’t break the application, Mistake for true difficulties, and FATAL in the event the technique can’t continue on.
Keep away from flooding your logs with extreme or irrelevant data. Far too much logging can obscure significant messages and decelerate your method. Deal with critical activities, state improvements, input/output values, and important determination points as part of your code.
Format your log messages Evidently and persistently. Consist of context, which include timestamps, request IDs, and performance names, so it’s simpler to trace issues in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to keep track of how variables evolve, what circumstances are achieved, and what branches of logic are executed—all with out halting This system. They’re Particularly precious in manufacturing environments wherever stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. With a properly-assumed-out logging strategy, you may reduce the time it will take to identify challenges, acquire deeper visibility into your apps, and Increase the General maintainability and dependability within your code.
Think Just like a Detective
Debugging is not simply a technological task—it's a kind of investigation. To proficiently identify and repair bugs, developers have to solution the procedure like a detective solving a mystery. This attitude can help stop working elaborate problems into manageable elements and observe clues logically to uncover the foundation induce.
Start by gathering evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or functionality troubles. The same as a detective surveys a criminal offense scene, accumulate just as much applicable information as you can with out jumping to conclusions. Use logs, test cases, and person experiences to piece alongside one another a transparent photo of what’s occurring.
Up coming, kind hypotheses. Request your self: What might be creating this behavior? Have any changes a short while ago been built to your codebase? Has this situation transpired prior to underneath very similar situation? The purpose will be to slender down alternatives and establish likely culprits.
Then, take a look at your theories systematically. Try and recreate the trouble inside a managed natural environment. When you suspect a particular perform or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, check with your code inquiries and Permit the outcomes lead you nearer to the reality.
Fork out near focus to little aspects. Bugs normally cover while in the least envisioned areas—similar to a missing semicolon, an off-by-one mistake, or even a race ailment. Be extensive and individual, resisting the urge to patch The difficulty with no fully knowledge it. Short-term fixes may perhaps conceal the real dilemma, just for it to resurface later.
Last of all, preserve notes on Anything you experimented with and learned. Just as detectives log their investigations, documenting your debugging system can conserve time for long run problems and support Other people have an understanding of your reasoning.
By pondering just like a detective, builders can sharpen their analytical abilities, technique complications methodically, and grow to be simpler at uncovering concealed issues in sophisticated devices.
Write Tests
Composing assessments is among the best solutions to improve your debugging abilities and Total progress performance. Tests not just support capture bugs early and also function a security Web that offers you confidence when making adjustments in your codebase. A properly-examined application is simpler to debug as it lets you pinpoint particularly wherever and when a dilemma takes place.
Get started with device assessments, which target specific features or modules. These modest, isolated assessments can speedily reveal no matter whether a particular piece of logic is Operating as expected. When a exam fails, you straight away know wherever to glance, appreciably minimizing time invested debugging. Unit checks are In particular valuable for catching regression bugs—troubles that reappear soon after Formerly getting set.
Next, combine integration assessments and stop-to-end checks into your workflow. These enable be certain that numerous parts of your software perform together efficiently. They’re specifically helpful for catching bugs that manifest in intricate methods with various parts or solutions interacting. If a little something breaks, your assessments can tell you which Component of the pipeline failed and underneath what situations.
Crafting exams also forces you to definitely Consider critically about your code. To check a feature adequately, you will need to be familiar with its inputs, anticipated outputs, and edge cases. This amount of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a strong starting point. Once the examination fails consistently, it is possible to deal with fixing the bug and look at your exam pass when The problem is fixed. This approach makes sure that the exact same bug doesn’t return in the future.
In a nutshell, producing checks turns debugging from a aggravating guessing sport into a structured and predictable approach—encouraging you catch much more bugs, more rapidly plus much more reliably.
Take Breaks
When debugging a tricky concern, it’s effortless to be immersed in the condition—staring at your display for hrs, striving Option just after solution. But Probably the most underrated debugging resources is just stepping away. Using breaks will help you reset your brain, lessen annoyance, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for way too very long, cognitive exhaustion sets in. You would possibly get started overlooking noticeable faults or misreading code that you wrote just several hours earlier. In this point out, your Mind becomes less efficient at problem-resolving. A brief stroll, a coffee break, or even switching to a different endeavor for ten–quarter-hour can refresh your target. Several developers report finding the foundation of a challenge once they've taken time for you to disconnect, letting their subconscious do the job from the track record.
Breaks also assist prevent burnout, Primarily through more time debugging sessions. Sitting down in front of a screen, mentally trapped, is not just unproductive but also draining. Stepping absent permits you to return with renewed energy in addition to a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a good guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–10 moment break. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, especially beneath limited deadlines, nevertheless it basically results in a lot quicker and more effective debugging Ultimately.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart approach. It presents your brain Place to breathe, improves your viewpoint, and allows you avoid the tunnel eyesight that often blocks your progress. Debugging is usually a mental puzzle, and relaxation is an element of solving it.
Find out From Each individual Bug
Each bug you come across is a lot more than simply a temporary setback—It really is a possibility to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can teach you some thing worthwhile when you take the time to reflect and evaluate what went Mistaken.
Start off by inquiring yourself a couple of crucial queries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with better practices like unit testing, code critiques, or logging? The answers usually reveal blind places as part of your workflow or being familiar with and help you build stronger coding habits going ahead.
Documenting bugs can even be an outstanding practice. Retain a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and what you acquired. Eventually, you’ll begin to see designs—recurring concerns or frequent errors—that you can proactively avoid.
In workforce environments, sharing That which you've uncovered from a read more bug together with your friends is often Specially potent. Whether or not it’s via a Slack concept, a short generate-up, or a quick understanding-sharing session, encouraging Some others avoid the same challenge boosts group performance and cultivates a more robust Studying society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as important aspects of your advancement journey. After all, a lot of the ideal builders will not be those who compose fantastic code, but individuals who continuously understand from their mistakes.
Ultimately, Each individual bug you resolve provides a brand new layer on your skill set. So upcoming time you squash a bug, take a second to mirror—you’ll occur away a smarter, far more able developer due to it.
Summary
Improving upon your debugging expertise can take time, practice, and persistence — although the payoff is huge. It helps make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a possibility to be better at Everything you do.